Create array sequence `[0, 1, ..., N-1]` in one line
Here are two compact code sequences to generate the N
-element array [0, 1, ..., N-1]
:
Solution 1 (requires ES5)
Array.apply(null, {length: N}).map(Function.call, Number);
Brief explanation
Array.apply(null, {length: N})
returns anN
-element array filled withundefined
(i.e.A = [undefined, undefined, ...]
).A.map(Function.call, Number)
returns anN
-element array, whose indexI
gets the result ofFunction.call.call(Number, undefined, I, A)
Function.call.call(Number, undefined, I, A)
collapses intoNumber(I)
, which is naturallyI
.- Result:
[0, 1, ..., N-1]
.
For a more thorough explanation, go here.
Solution 2 (requires ES6)
It uses Array.from
https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_Objects/Array/from
Array.from(new Array(N),(val,index)=>index);
Solution 3 (requires ES6)
Array.from(Array(N).keys());
Brief explanation
A = new Array(N)
returns an array withN
holes (i.e.A = [,,,...]
, butA[x] = undefined
forx
in0...N-1
).F = (val,index)=>index
is simplyfunction F (val, index) { return index; }
Array.from(A, F)
returns anN
-element array, whose indexI
gets the results ofF(A[I], I)
, which is simplyI
.- Result:
[0, 1, ..., N-1]
.
One More Thing
If you actually want the sequence [1, 2, …, N], Solution 1 becomes:
Array.apply(null, {length: N}).map(function(value, index){
return index + 1;
});
and Solution 2:
Array.from(new Array(N),(val,index)=>index+1);
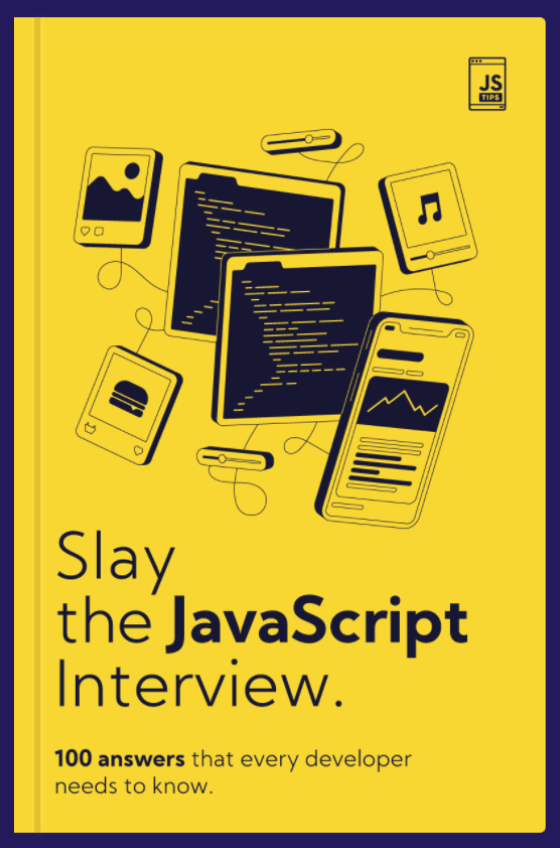
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
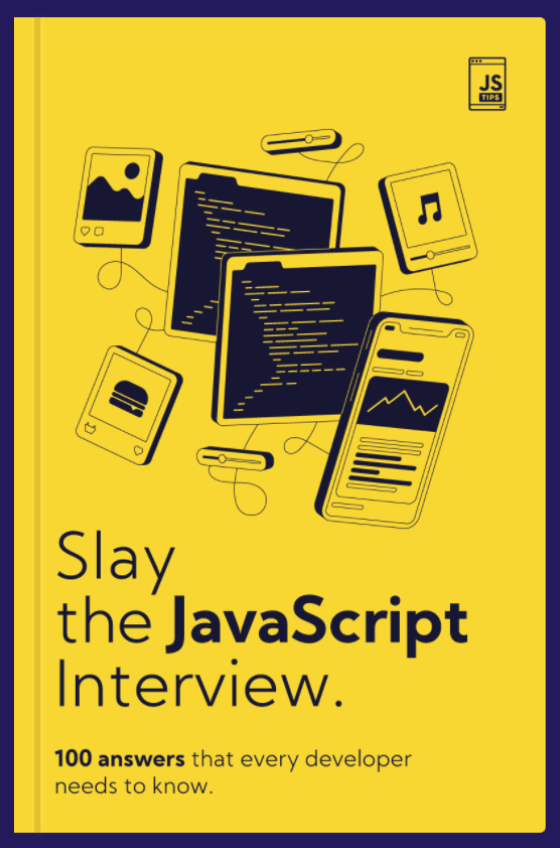
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW