Arrays multidimensionales en JavaScript
Estas son las tres formas conocidas para fusionar arrays multidimensional en una sola arrays.
Dado el array:
var myArray = [[1, 2],[3, 4, 5], [6, 7, 8, 9]];
Queremos tener este resultado:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
Solucion 1: usando concat()
y apply()
var myNewArray = [].concat.apply([], myArray);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
Solucion 2: usando reduce()
var myNewArray = myArray.reduce(function(prev, curr) {
return prev.concat(curr);
});
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
Solucion 3:
var myNewArray3 = [];
for (var i = 0; i < myArray.length; ++i) {
for (var j = 0; j < myArray[i].length; ++j)
myNewArray3.push(myArray[i][j]);
}
console.log(myNewArray3);
// [1, 2, 3, 4, 5, 6, 7, 8, 9]
Echar un vistazo here estos 3 algoritmos en accion.
Para array infinitamente anidado probar Underscore flatten().
Si tiene curiosidad por la performance, here.
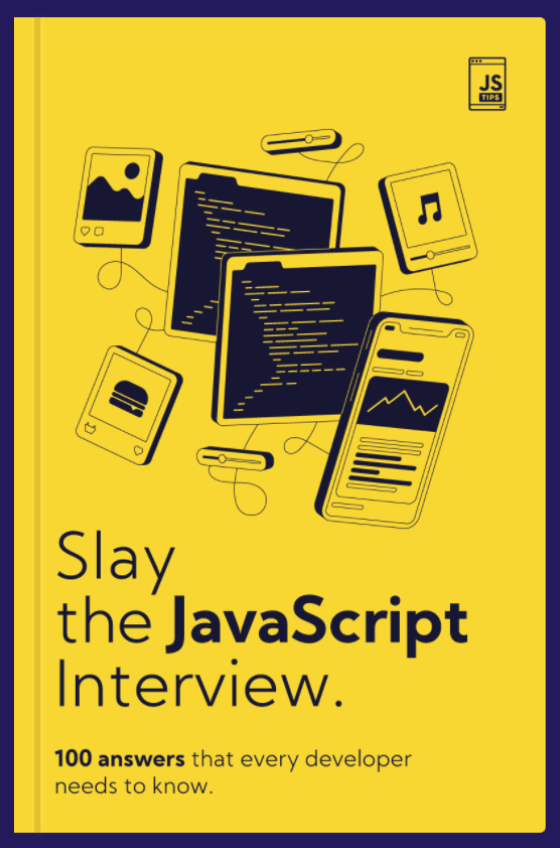
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
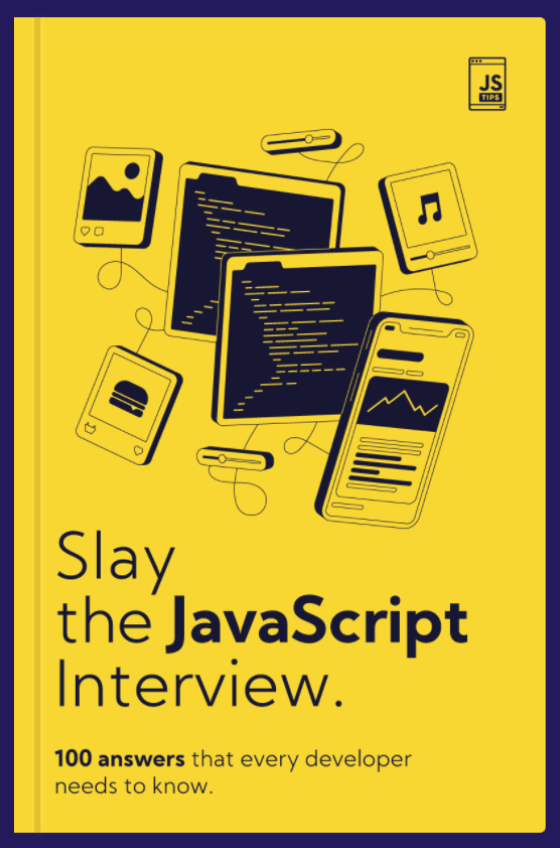
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW