Obtener extensión de archivo
Pregunta: ¿Cómo conseguir la extensión de archivo?
var file1 = "50.xsl";
var file2 = "30.doc";
getFileExtension(file1); //returs xsl
getFileExtension(file2); //returs doc
function getFileExtension(filename) {
/*TODO*/
}
Solucion 1: Expresion regular
function getFileExtension1(filename) {
return (/[.]/.exec(filename)) ? /[^.]+$/.exec(filename)[0] : undefined;
}
Solucion 2: Metodo split
function getFileExtension2(filename) {
return filename.split('.').pop();
}
Estas dos soluciones no podían manejar algunos casos extremos, aquí hay otra solución más robusta.
Solucion 3: Metodos slice
, lastIndexOf
function getFileExtension3(filename) {
return filename.slice((filename.lastIndexOf(".") - 1 >>> 0) + 2);
}
console.log(getFileExtension3('')); // ''
console.log(getFileExtension3('filename')); // ''
console.log(getFileExtension3('filename.txt')); // 'txt'
console.log(getFileExtension3('.hiddenfile')); // ''
console.log(getFileExtension3('filename.with.many.dots.ext')); // 'ext'
¿Como funciona?
- String.lastIndexOf() el método devuelve la última aparición del valor especificado (
'.'
en este caso). Devuelve-1
si no se encuentra el valor. - Los valores de retorno de
lastIndexOf
para el parámetro'filename'
y'.hiddenfile'
son0
y-1
respectivamente.Zero-fill operador de desplazamiento a la derecha (»>) transformará-1
a4294967295
y-2
a4294967294
, aquí es un truco para asegurar el nombre del archivo sin cambios en esos casos extremos. - String.prototype.slice() extrae la extensión del índice que se calculó anteriormente. Si el índice es mayor que la longitud del nombre de archivo, el resultado es
""
.
Comparación
Solucion | Parametros | Resultados |
---|---|---|
Solucion 1: Expresion regular | ’‘ ‘filename’ ‘filename.txt’ ‘.hiddenfile’ ‘filename.with.many.dots.ext’ |
undefined undefined ‘txt’ ‘hiddenfile’ ‘ext’ |
Solucion 2: Metodo split |
’‘ ‘filename’ ‘filename.txt’ ‘.hiddenfile’ ‘filename.with.many.dots.ext’ |
’’ ‘filename’ ‘txt’ ‘hiddenfile’ ‘ext’ |
Solucion 3: Metodos slice , lastIndexOf |
’‘ ‘filename’ ‘filename.txt’ ‘.hiddenfile’ ‘filename.with.many.dots.ext’ |
’’ ‘’ ‘txt’ ‘’ ‘ext’ |
Demo de performance
Here es la demostración de los códigos anteriores.
Here es la prueba de rendimiento de esos 3 soluciones.
Codigo
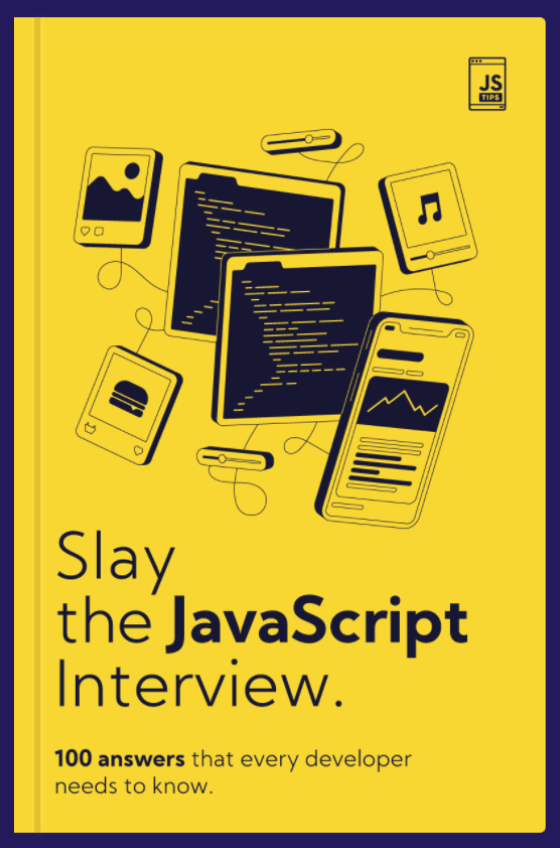
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
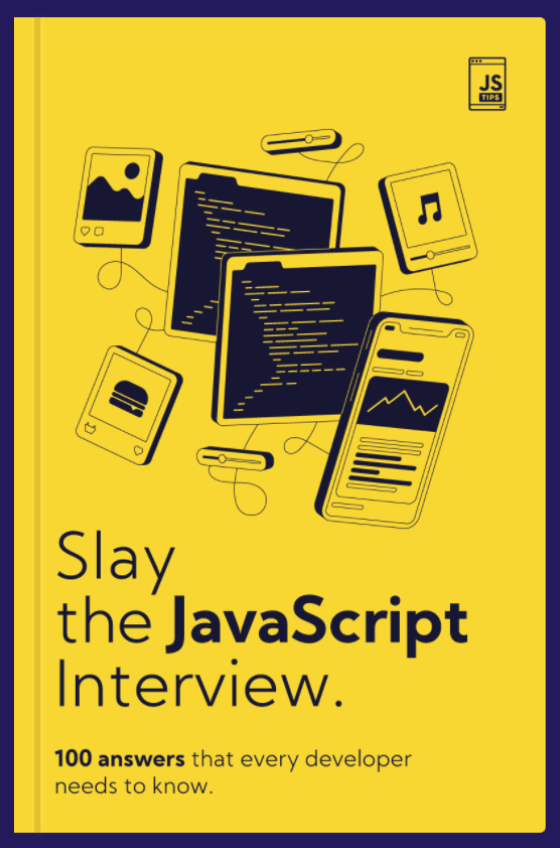
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW