Assignment Operators
Assigning is very common. Sometimes typing becomes time consuming for us ‘Lazy programmers’. So, we can use some tricks to help us and make our code cleaner and simpler.
This is the similar use of
x += 23; // x = x + 23;
y -= 15; // y = y - 15;
z *= 10; // z = z * 10;
k /= 7; // k = k / 7;
p %= 3; // p = p % 3;
d **= 2; // d = d ** 2;
m >>= 2; // m = m >> 2;
n <<= 2; // n = n << 2;
n ++; // n = n + 1;
n --; n = n - 1;
++
and --
operators
There is a special ++
operator. It’s best to explain it with an example:
var a = 2;
var b = a++;
// Now a is 3 and b is 2
The a++
statement does this:
- return the value of
a
- increment
a
by 1
But what if we wanted to increment the value first? It’s simple:
var a = 2;
var b = ++a;
// Now both a and b are 3
See? I put the operator before the variable.
The --
operator is similar, except it decrements the value.
If-else (Using ternary operator)
This is what we write on regular basis.
var newValue;
if(value > 10)
newValue = 5;
else
newValue = 2;
We can user ternary operator to make it awesome:
var newValue = (value > 10) ? 5 : 2;
Null, Undefined, Empty Checks
if (variable1 !== null || variable1 !== undefined || variable1 !== '') {
var variable2 = variable1;
}
Shorthand here:
var variable2 = variable1 || '';
P.S.: If variable1 is a number, then first check if it is 0.
Object Array Notation
Instead of using:
var a = new Array();
a[0] = "myString1";
a[1] = "myString2";
Use this:
var a = ["myString1", "myString2"];
Associative array
Instead of using:
var skillSet = new Array();
skillSet['Document language'] = 'HTML5';
skillSet['Styling language'] = 'CSS3';
Use this:
var skillSet = {
'Document language' : 'HTML5',
'Styling language' : 'CSS3'
};
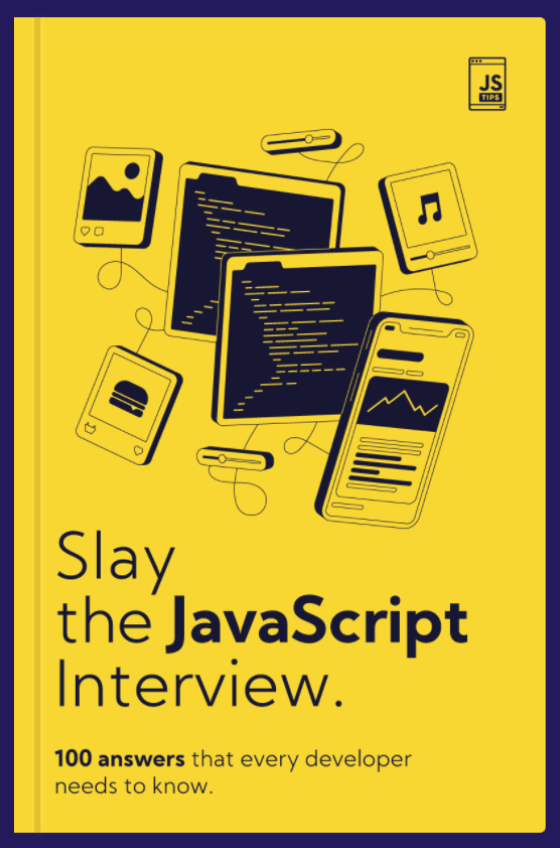
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
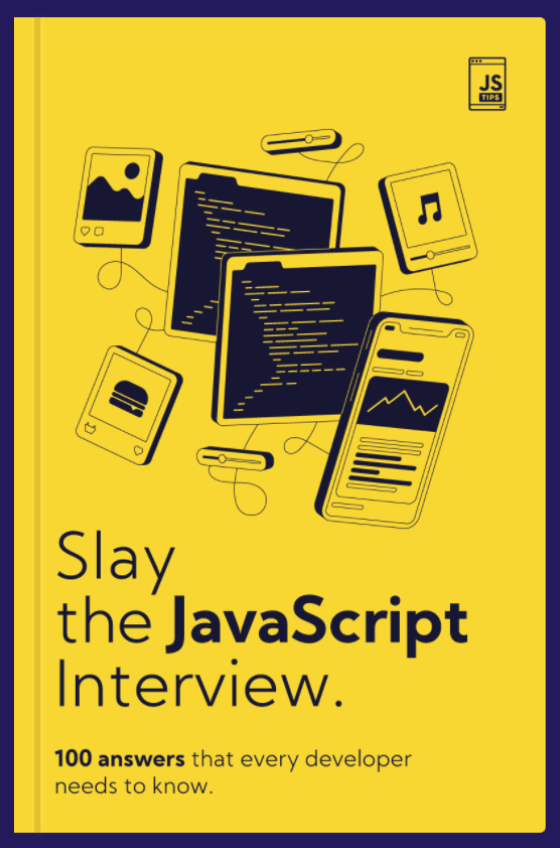
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW