Check if a property is in a Object
When you have to check if a property is present in an object, you probably are doing something like this:
var myObject = {
name: '@tips_js'
};
if (myObject.name) { ... }
That’s ok, but you have to know that there are two native ways for this kind of thing, the in
operator and Object.hasOwnProperty
. Every object descended from Object
, has both ways available.
See the big Difference
var myObject = {
name: '@tips_js'
};
myObject.hasOwnProperty('name'); // true
'name' in myObject; // true
myObject.hasOwnProperty('valueOf'); // false, valueOf is inherited from the prototype chain
'valueOf' in myObject; // true
Both differ in the depth at which they check the properties. In other words, hasOwnProperty
will only return true if key is available on that object directly. However, the in
operator doesn’t discriminate between properties created on an object and properties inherited from the prototype chain.
Here’s another example:
var myFunc = function() {
this.name = '@tips_js';
};
myFunc.prototype.age = '10 days';
var user = new myFunc();
user.hasOwnProperty('name'); // true
user.hasOwnProperty('age'); // false, because age is from the prototype chain
Check the live examples here!
I also recommend reading this discussion about common mistakes made when checking a property’s existence in objects.
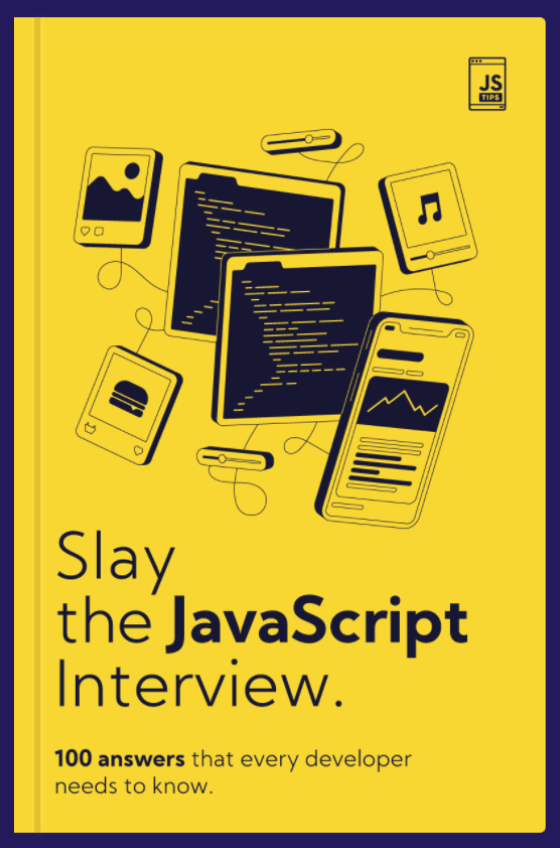
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
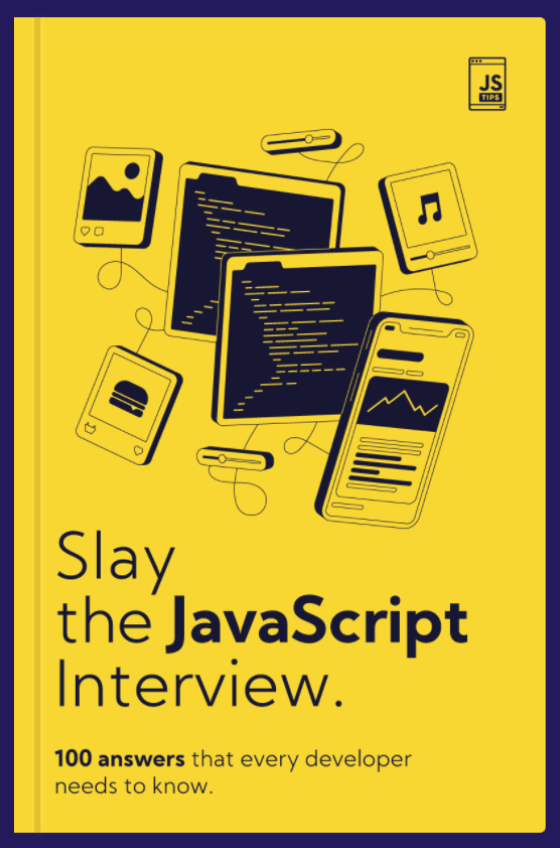
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW