Comma operator in JS
Apart from being just a delimiter, the comma operator allows you to put multiple statements in a place where one statement is expected. Eg:-
for(var i=0, j=0; i<5; i++, j++, j++){
console.log("i:"+i+", j:"+j);
}
Output:-
i:0, j:0
i:1, j:2
i:2, j:4
i:3, j:6
i:4, j:8
When placed in an expression, it evaluates every expression from left to right and returns the right most expression.
Eg:-
function a(){console.log('a'); return 'a';}
function b(){console.log('b'); return 'b';}
function c(){console.log('c'); return 'c';}
var x = (a(), b(), c());
console.log(x); // Outputs "c"
Output:-
"a"
"b"
"c"
"c"
- Note: The comma(
,
) operator has the lowest priority of all javascript operators, so without the parenthesis the expression would become:(x = a()), b(), c();
.
Playground
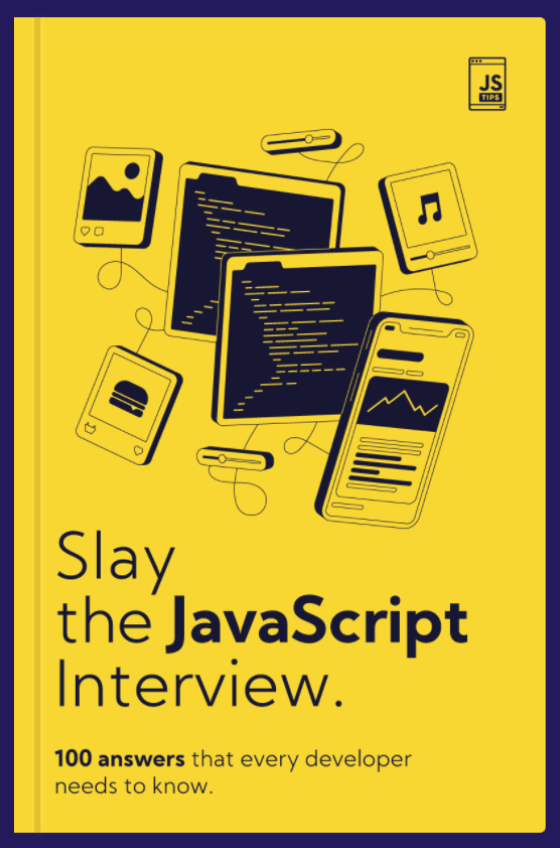
MEET THE NEW JSTIPS BOOK
You no longer need 10+ years of experience to get your dream job.
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
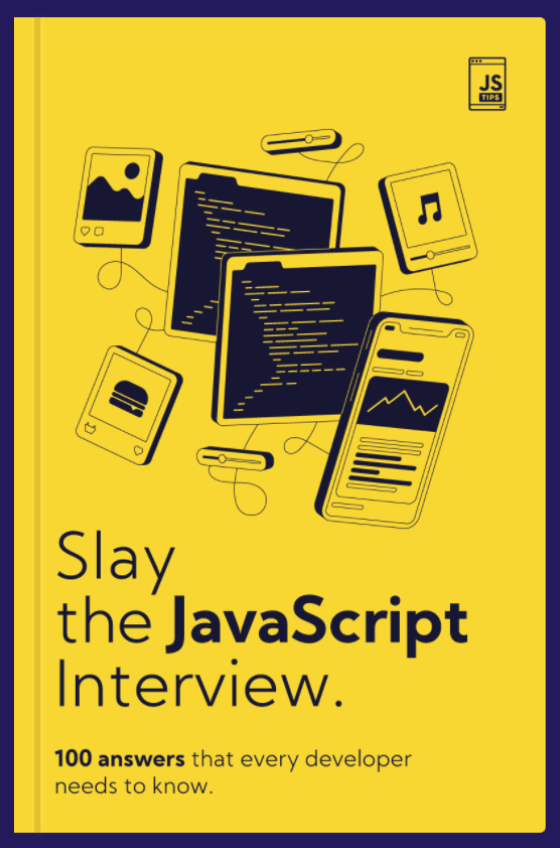
MEET THE NEW JSTIPS BOOK
The book to ace the JavaScript Interview.
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW