Map() to the rescue; adding order to Object properties
Object properties order
An object is a member of the type Object. It is an unordered collection of properties each of which contains a primitive value, object, or function. A function stored in a property of an object is called a method. ECMAScript
Take a look in action
var myObject = {
z: 1,
'@': 2,
b: 3,
1: 4,
5: 5
};
console.log(myObject) // Object {1: 4, 5: 5, z: 1, @: 2, b: 3}
for (item in myObject) {...
// 1
// 5
// z
// @
// b
Each browser have his own rules about the order in objects bebause technically, order is unspecified.
How to solve this?
Map
Using a new ES6 feature called Map. A Map object iterates its elements in insertion order — a for...of
loop returns an array of [key, value] for each iteration.
var myObject = new Map();
myObject.set('z', 1);
myObject.set('@', 2);
myObject.set('b', 3);
for (var [key, value] of myObject) {
console.log(key, value);
...
// z 1
// @ 2
// b 3
Hack for old browsers
Mozilla suggest:
So, if you want to simulate an ordered associative array in a cross-browser environment, you are forced to either use two separate arrays (one for the keys and the other for the values), or build an array of single-property objects, etc.
// Using two separate arrays
var objectKeys = [z, @, b, 1, 5];
for (item in objectKeys) {
myObject[item]
...
// Build an array of single-property objects
var myData = [{z: 1}, {'@': 2}, {b: 3}, {1: 4}, {5: 5}];
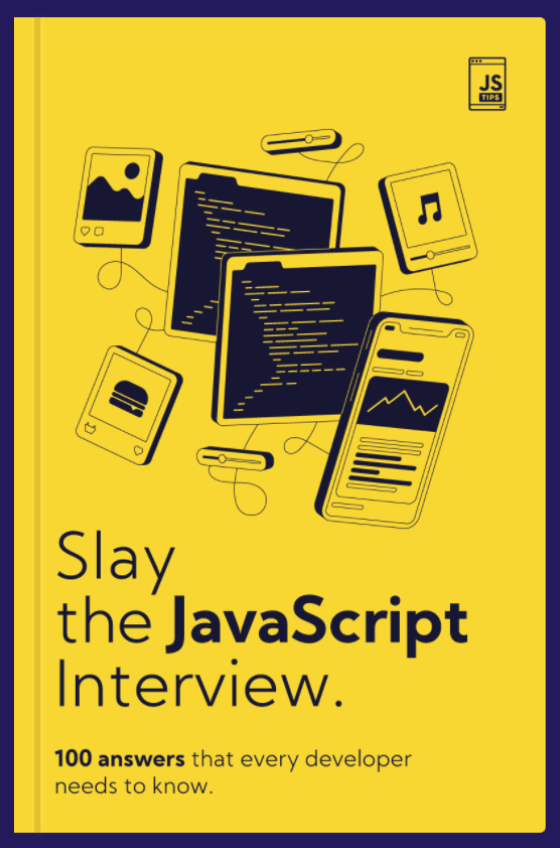
Use the 100 answers in this short book to boost your confidence and skills to ace the interviews at your favorite companies like Twitter, Google and Netflix.
GET THE BOOK NOW
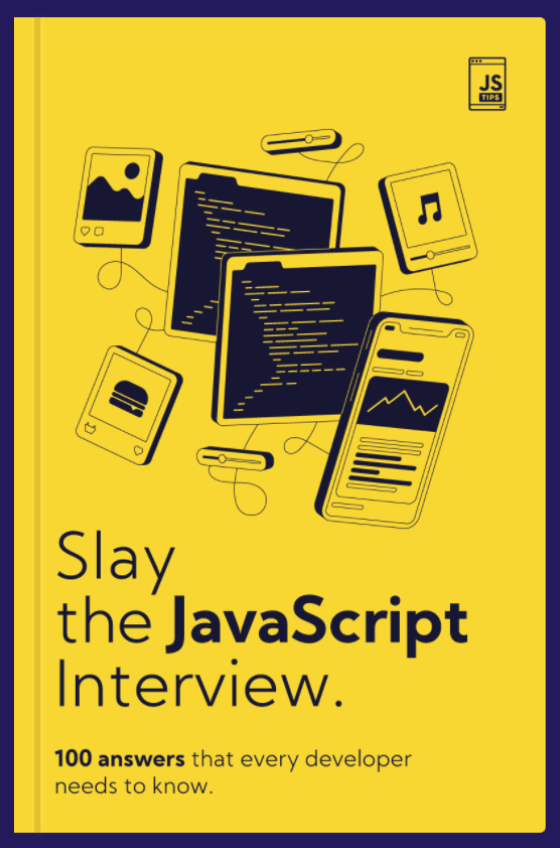
A short book with 100 answers designed to boost your knowledge and help you ace the technical interview within a few days.
GET THE BOOK NOW